Send email Django
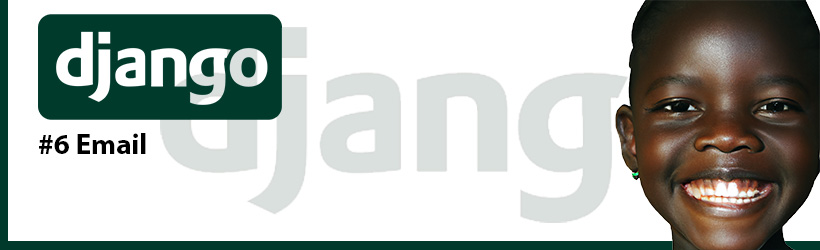
Send an e-mail
For this, the following lines must be added at the end of the 'settings.py' file:
EMAIL_HOST="smtp.gmail.com"
EMAIL_USE_TLS=True
EMAIL_PORT=587
EMAIL_HOST_USER="fernandocarrillos86@gmail.com"
EMAIL_HOST_PASSWORD="mypassword"
In case you want to test this connection, you can enter the following lines from the console:
python manage.py shell
from django.core.mail import send_mail
send_mail('Subject here','Here is the message','whosend@gmail.com',['whoreceive@hotmail.com'],fail_silently=False)
Create a view that receives the information coming from a form and executes the submission:
from django.shortcuts import render, redirect
from Contact.forms import Contact_Form
from django.core.mail import EmailMessage
# Create your views here.
def Load_Form(request):
Form=Contact_Form()
if request.method=="POST":
Response_Form=Contact_Form(data=request.POST)
if Response_Form.is_valid():
Name=request.POST.get("Name")
Email=request.POST.get("Email")
Content=request.POST.get("Content")
email=EmailMessage(
"Mensaje desde App DJAngo",
"El usuario con nombre {} con la dirección {} escribe lo siguiente: {}".format(Name,Email,Content),
"",
['fernandocarrillos86@gmail.com'],
reply_to=[Email]
)
try:
email.send()
return redirect("/contact?valid")
except:
return redirect("/contact?novalid")
return render(request, "Contact/contact.html",{'Form':Form})
Template HTML:
Template HTML:
{% block content %}
{% if 'valid' in request.GET %}
The data was loaded successfully
{% endif %}
{% if 'novalid' in request.GET %}
The data wasn't loaded successfully
{% endif %}
{% endblock %}
Thanks for reading :)
I invite you to continue reading other entries and visiting us again soon.