ORM
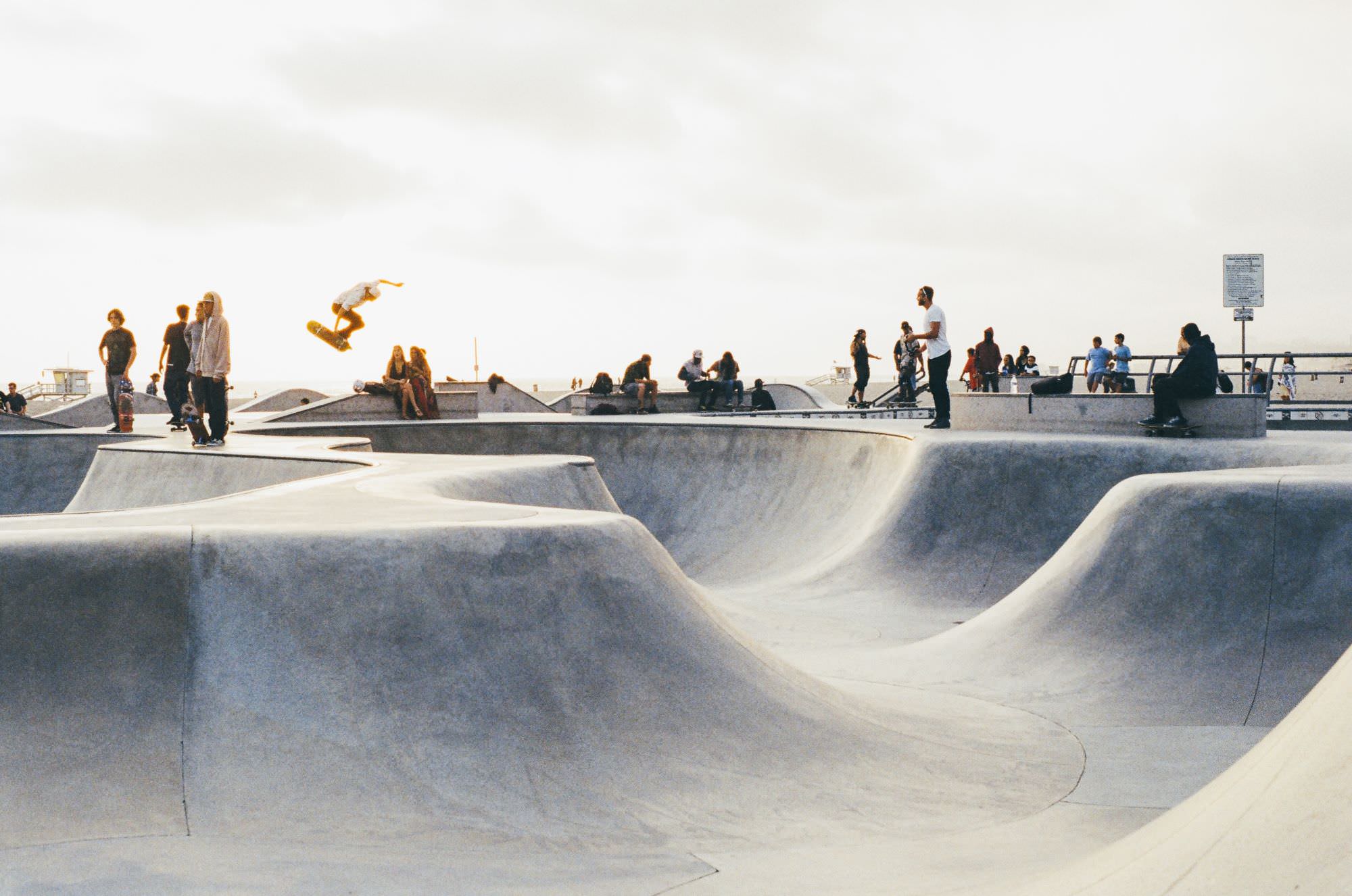
ORM Django
Let's create a small project for practicing the handling data using the ORM offered by Django.
-
Create a virtual enviroment and active it:
path-to-the-project>py -m venv env path-to-the-project\env\Scripts>activate (env) path-to-the-project\env\Scripts>
-
Install Django:
(env) path-to-the-project>pip install django
-
Create a project:
(env) path-to-the-project>django-admin startproject learning_django
-
Run the server:
(env) path-to-the-project\learning_django>py manage.py runserver
At the moment we can get this:
-
Create an app called ORM and register it in learning_django/settings.py:
path-to-the-project\learning_django>py manage.py startapp orm
# Application definition INSTALLED_APPS = [ 'orm', # Register the app orm 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', ]
-
Register the Templates and Static directories:
We are going to use some HTML templates, then let's register the "templates" directory and the "static" directory at learning_django/settings.py and create "templates" directory at project's root and "orm/templates" directory.
# Templates TEMPLATES = [ { 'BACKEND': 'django.template.backends.django.DjangoTemplates', 'DIRS': ['templates'], 'APP_DIRS': True, 'OPTIONS': { 'context_processors': [ 'django.template.context_processors.debug', 'django.template.context_processors.request', 'django.contrib.auth.context_processors.auth', 'django.contrib.messages.context_processors.messages', ], }, }, ]
Static directory:
# Static directory STATIC_URL = 'static/' STATICFILES_DIRS = ['static', ]
-
Set the connection to Database:
In this case, I am going to use a MariaDB database, this is set at learning_django/settings.py:
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.mysql', 'NAME': 'django_orm', 'USER': 'root', 'PASSWORD': '', 'HOST': 'localhost', 'PORT': '3306', } }
-
Create the database:
Create a database called "django_orm", the tables will be created by migrations. In this example we are using MySQL or MariaDB.
-
Install the driver for connecting to MySQL Databases:
(env) path-to-the-project\learning_django>pip install mysqlclient
-
Create the following views in orm/views.py:
from django.shortcuts import render from django.http import HttpResponse # Create your views here. def sql_where(request): return HttpResponse('where') def sql_insert(request): return HttpResponse('insert') def sql_update(request): return HttpResponse('update') def sql_delete(request): return HttpResponse('delete') def sql_join(request): return HttpResponse('join')
-
Create the "orm/urls.py" file and register the urls for the views:
from django.urls import path from . import views app_name = 'orm' # namespace for app's urls urlpatterns = [ # path(url,function,name for this url) # ex: /orm/insert path('insert', views.sql_insert, name='insert'), path('where', views.sql_where, name='where'), path('update', views.sql_update, name='update'), path('delete', views.sql_delete, name='delete'), path('join', views.sql_join, name='join'), ]
-
Include the ORM's urls in the project's urls:
Set the file learning_django/urls.py:
from django.contrib import admin from django.urls import path, include urlpatterns = [ path('orm/', include('orm.urls')), path('admin/', admin.site.urls), ]
At this moment we can run the server to get this:
(env) path-to-the-project\learning_django>py manage.py runserver
and the same for where, delete and join.
-
Create the models:
At orm/models set:
from django.db import models # Create your models here. class Country(models.Model): id = models.AutoField(primary_key=True) country = models.CharField(max_length=255,unique=True) class Department(models.Model): department = models.CharField(max_length=255,null=False,unique=True) class Product(models.Model): product = models.CharField(max_length=255) origin = models.ForeignKey(Country,null=True,on_delete=models.SET_NULL) department=models.ManyToManyField(Department) price = models.FloatField(null=False,blank=False)
-
Make migrations:
(env) path-to-the-project/learn_django>py manage.py makemigrations orm
The console should answer:
Migrations for 'orm': orm\migrations\0001_initial.py - Create model Country - Create model Department - Create model Product
For whatching the SQL changes taht will be executed:
(env) path-to-the-project/learn_django>py manage.py sqlmigrate orm 0001
The console should answer:
-- -- Create model Country -- CREATE TABLE `orm_country` (`id` integer AUTO_INCREMENT NOT NULL PRIMARY KEY, `country` varchar(255) NOT NULL UNIQUE); -- -- Create model Department -- CREATE TABLE `orm_department` (`id` bigint AUTO_INCREMENT NOT NULL PRIMARY KEY, `department` varchar(255) NOT NULL UNIQUE); -- -- Create model Product -- CREATE TABLE `orm_product` (`id` bigint AUTO_INCREMENT NOT NULL PRIMARY KEY, `product` varchar(255) NOT NULL, `price` double precision NOT NULL, `origin_id` integer NULL); CREATE TABLE `orm_product_department` (`id` bigint AUTO_INCREMENT NOT NULL PRIMARY KEY, `product_id` bigint NOT NULL, `department_id` bigint NOT NULL); ALTER TABLE `orm_product` ADD CONSTRAINT `orm_product_origin_id_37ac325a_fk_orm_country_id` FOREIGN KEY (`origin_id`) REFERENCES `orm_country` (`id`); ALTER TABLE `orm_product_department` ADD CONSTRAINT `orm_product_department_product_id_department_id_fa4e7392_uniq` UNIQUE (`product_id`, `department_id`); ALTER TABLE `orm_product_department` ADD CONSTRAINT `orm_product_department_product_id_a654837b_fk_orm_product_id` FOREIGN KEY (`product_id`) REFERENCES `orm_product` (`id`); ALTER TABLE `orm_product_department` ADD CONSTRAINT `orm_product_departme_department_id_eb5711f7_fk_orm_depar` FOREIGN KEY (`department_id`) REFERENCES `orm_department` (`id`);
Finally, excecute the migration:
(env) path-to-the-project/learn_django>py manage.py migrate
If we check the database we should find some tables were created. Some of them are from models built-in in Django and there are some tables which name begin with "orm_", those last were created for our ORM model.
Thanks for reading :)
I invite you to continue reading other entries and visiting us again soon.