Backend section
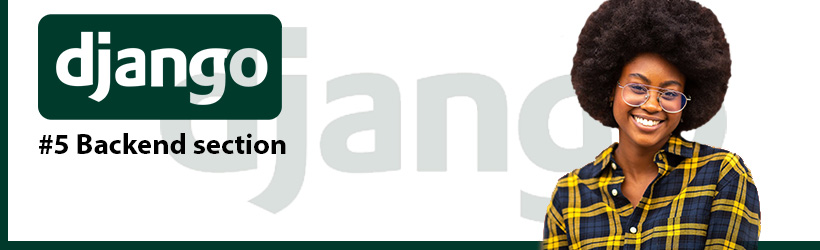
Create a superUser
From the console, enter the following command and complete the requested data
(env) path-to-the-project>\my_project>python manage.py createsuperuser
Later, it is necessary to enter username, email and password:
Username (leave blank to use 'ferna'): fer
Email address: fer@fernandoc.dev
Password:
Password (again):
The terminal answer the following:
Superuser created successfully.
Now, run the server and visit "localhost:8000/admin"
(env) path-to-the-project>\my_project>python manage.py runserver
Enter the credentials of the user just created
Add CRUD of a model (table) to the admin panel
In the 'admin.py' file of the application, the following code must be added:
from django.contrib import admin
from My_App.models import My_table
# Register your models here.
admin.site.register(My_table)
Change the headers of the Administration CRUD, without affecting the DB
In order for the CRUD to show a header other than the field name, the 'verbose_name' attribute must be defined in the model in that field. This should be done in the application's 'models.py' file:
class My_table(models.Model):
field1=models.CharField(max_length=30, verbose_name='Name')
If you want to add or remove columns from the table, so that they are shown in the CRUD, for this you must create a new class, which inherits from the original class of the model. This should be done in the application's 'admin.py' file:
from django.contrib import admin
from My_App.models import My_table
# Register your models here.
class New_My_table(admin.ModelAdmin):
list_display=('field1','field4')#We only want to print field1 and field4
admin.site.register(My_table,New_My_table)
Add a search field to filter the CRUD
For this, a new class must be created, which inherits from the original class of the model. This should be done in the application's 'admin.py' file:
from django.contrib import admin
from My_App.models import My_table
# Register your models here.
class New_My_table(admin.ModelAdmin):
search_fields=('field1','field3')#We only want to search items using field1 and 3 like filters
admin.site.register(My_table,New_My_table)
Add a filter column for the CRUD
For this, a new class must be created, which inherits from the original class of the model. This should be done in the application's 'admin.py' file:
class New_My_table(admin.ModelAdmin):
list_filter=('field2',)#The filter will work using the field2
admin.site.register(My_table,New_My_table)
Allow automatic datetime fields to be visible in the CRUD
For this, a new class must be created, which inherits from the original class of the model. This should be done in the application's 'admin.py' file:
class Activate_Datetime_Fields(admin.ModelAdmin):
readonly_fields=('field2', 'field3')#The datetime fields with auto_now_add=True feature
#are not visible until this configuration
admin.site.register(My_table, Activate_Datetime_Fields)
Change the language of the administration panel
In the 'settings.py' file, in the 'LANGUAGE_CODE' variable, place 'es-eu' for Spanish.
Thanks for reading :)
I invite you to continue reading other entries and visiting us again soon.