Models Django
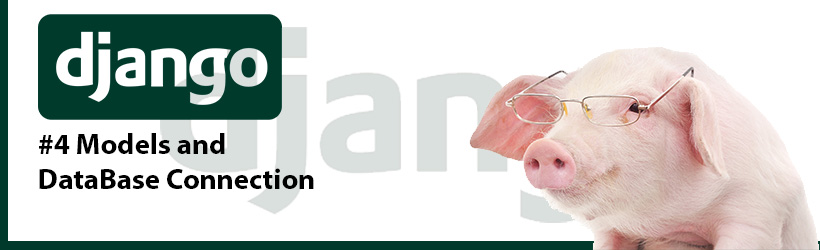
DataBase Connection
At "crud/settings.py" you can set the DataBase Connection, the following is an example for PostgreSQL:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': 'mydatabase',
'USER': 'mydatabaseuser',
'PASSWORD': 'mypassword',
'HOST': '127.0.0.1',
'PORT': '5432',
}
}
Examples for ENGINE values according to the DBMS:
SQLite3: 'django.db.backends.sqlite3'
PostgreSQL: 'django.db.backends.postgresql'
MySQL/MariaDB: 'django.db.backends.mysql'
Oracle: 'django.db.backends.oracle'
In this case you must set the next values for using MariaDB running on localhost:3306 with a DataBase called "django":
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'django',
'USER': 'root',
'PASSWORD': '',
'HOST': 'localhost',
'PORT': '3306',
}
}
It is important to install the driver according to the DBMS, in this example we are going to use MySQL, then we must install "mysqlclient" driver:
(env) path-to-the-project/my_project>pip install mysqlclient
Finally, the following command will execute the changes in the DataBase:
(env) path-to-the-project/my_project>python manage.py migrate
Create Models
All my_app's models will be in a unique file: "my_app/models.py":
from django.db import models
class Role(models.Model):
role = models.CharField(max_length=255)
class User(models.Model):
id = models.AutoField(primary_key=True)
name = models.CharField(max_length=255)
username = models.CharField(max_length=255)
birthday = models.DateField()
role = models.ForeignKey(Role, on_delete=models.CASCADE)
active = models.BooleanField(default=True)
Django creates the entities in the DataBase according to the declared Models
Finally, you must use three commands:
1. For indicating there are changes in the my_app model and must be apply on migrations:
(env) path-to-the-project/my_project>py manage.py makemigrations my_app
The termial answer this:
Migrations for 'my_app':
my_app\migrations\0001_initial.py
- Create model Role
- Create model User
2. For whatching the SQL changes taht will be executed:
(env) path-to-the-project/my_project>py manage.py sqlmigrate my_app 0001
The terminal answer:
--
-- Create model Role
--
CREATE TABLE `my_app_role` (`id` bigint AUTO_INCREMENT NOT NULL PRIMARY KEY, `role` varchar(255) NOT NULL);
--
-- Create model User
--
CREATE TABLE `my_app_user` (`id` integer AUTO_INCREMENT NOT NULL PRIMARY KEY, `name` varchar(255) NOT NULL, `username` varchar(255) NOT NULL, `birthday` date NOT NULL, `active` bool NOT NULL, `role_id` bigint NOT NULL);
ALTER TABLE `my_app_user` ADD CONSTRAINT `my_app_user_role_id_d87f73e6_fk_my_app_role_id` FOREIGN KEY (`role_id`) REFERENCES `my_app_role` (`id`);
3. For executing the migration in the DataBase:
(env) path-to-the-project/my_project>py manage.py migrate
Now, the new tables according to the models, should be created.
Before finish this post, I would like to show a couple of queries done to ChatGPT while I make this article:
Django Data types
1. AutoField: IntegerField that automatically increments.
2. BigAutoField: BigIntegerField that automatically increments.
3. BinaryField: Binary data, up to 2^31 - 1 bytes.
4. BooleanField: Boolean (True/False) value.
5. CharField: Character string of a fixed length.
6. DateField: Date (year, month, day).
7. DateTimeField: Date and time.
8. DecimalField: Fixed-point decimal numbers.
9. DurationField: Time duration.
10. EmailField: Email address.
11. FileField: File path.
12. FilePathField: File path from a set of choices.
13. FloatField: Floating-point number.
14. ImageField: Image path.
15. IntegerField: Integer value.
16. GenericIPAddressField: IPv4 or IPv6 address.
17. NullBooleanField: Like a BooleanField, but allows NULL values.
18. PositiveIntegerField: Integer value greater than or equal to zero.
19. PositiveSmallIntegerField: Integer value greater than or equal to zero and less than 32768.
20. SlugField: Short label for URL use, containing only letters, numbers, hyphens or underscores.
21. SmallAutoField: SmallIntegerField that automatically increments.
22. SmallIntegerField: Integer value between -32768 and 32767.
23. TextField: Text of arbitrary length.
24. TimeField: Time of day.
25. URLField: URL.
Attributes
1. null: If True, Django will store empty values as NULL in the database. Default is False.
2. blank: If True, the field is allowed to be blank. Default is False.
3. choices: A sequence of 2-tuples where the first element is the value to be stored in the database and the second element is the human-readable name. Can be used to create drop-down select boxes in forms.
4. default: The default value for the field. Can be a value or a callable.
5. editable: If False, the field will not be displayed in the admin or any ModelForm. Default is True.
6. help_text: Extra "help" text to be displayed alongside the field in forms.
7. primary_key: If True, the field is the primary key for the model. Only one field in a model can have this attribute set to True.
8. unique: If True, the field must be unique throughout the table. Default is False.
9. verbose_name: A human-readable name for the field, used in the admin and other places where the field is displayed.
An example Model
This is a model declaration which uses the most common data types and attributes for holding like an example:
from django.db import models
class ExampleModel(models.Model):
name = models.CharField(max_length=100, unique=True, help_text='Enter the name of the object')
description = models.TextField(blank=True, help_text='Enter a brief description of the object')
is_active = models.BooleanField(default=True, help_text='Is this object currently active?')
timestamp = models.DateTimeField(auto_now_add=True, help_text='Date and time the object was created')
rating = models.DecimalField(max_digits=5, decimal_places=2, null=True, blank=True, help_text='The rating of the object')
image = models.ImageField(upload_to='images/', null=True, blank=True, help_text='Upload an image of the object')
Thanks for reading :)
I invite you to continue reading other entries and visiting us again soon.