Object-Oriented Programming
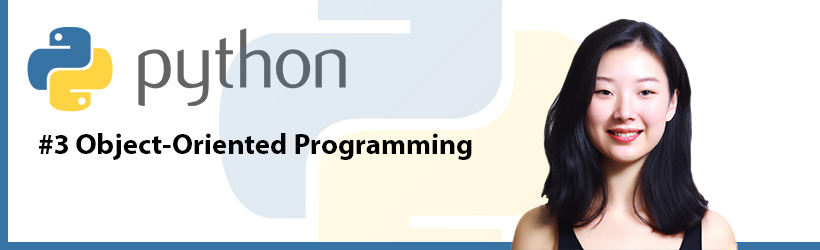
Class declaration
Declaration of an empty class
class my_empty_class: # Declaration of an empty class
pass
my_object = my_empty_class() # my_object is an object that instances the class my_empty_class
print(type(my_object))
#<class '__main__.my_empty_class'>
Declaration of a conventional class
class cars_factory:
# Builder method
def __init__(self, time, name=None, wheels=None):
# Atributes
self.time = time
self.name = name
self.wheels = wheels
if name is not None and wheels is not None:
print("It is expected the car {} will be built with {} wheels".format(name, wheels))
print(f"The car {self.name} was built succesfully")
else:
print("A car was built")
# Destructor method
def __del__(self):
print(f"The car {self.name} was destroyed")
# Special methods
def __str__(self):
return "The {} was built successfully, it took {} minutes and has {} wheels".format(self.name, self.time, self.wheels)
def __len__(self):
return self.time
# Methods
def my_method(self):
print(f"This car needs {self.wheels} wheels to work")
Object creation
my_car = cars_factory(5, 'Aveo', 10) # my_car is an object that instances the class factory
Find out what class an object belongs to
Type function
print(type(my_car))
# __main__.cars_factory
Isinstance function
isinstance(test, my_class) # Get back True/False
Issubclass function
issubclass(third_class, my_class)# Give back True/False
classes = (first_class, second_class, my_class)
issubclass(third_class, classes)# Give back True/False
Dir function
Se emplea para conocer todos los métodos y atributos de un objeto o de una clase
print(dir(cars_factory))
Declaration of attributes
There are several ways to declare attributes to the class:
1. Declaring the variable inside the class:
class cars_factory:
# Atributes
my_attribute = 'Fast engine'
2. Declaring the variable in the __init__ constructor method:
class cars_factory:
# Builder method
def __init__(self, time, name=None, wheels=None):
# Atributes
self.time = time
3. Declaring the variable outside the class, prepending the object
my_car.new_feature = 'Spoiler Activated'
__init__ constructor method
This function is executed when the object is created and is used to give value to its properties, as well as to carry out actions necessary for the initialization of the object. As the first argument, the keyword “self” (by convention) must always be declared, which refers to the current instance of the class and allows access to the properties of the object.
class cars_factory:
# Builder method
def __init__(self, time, name=None, wheels=None):
# Atributes
self.time = time
self.name = name
self.wheels = wheels
if name is not None and wheels is not None:
print("It is expected the car {} will be built with {} wheels".format(name, wheels))
print(f"The car {self.name} was built succesfully")
else:
print("A car was built")
Any other parameter could be used instead of “self”, and through this parameter the properties of the object would be accessed. The following example uses “my_object”
class cars_factory:
# Builder method
def __init__(my_object, time, name=None, wheels=None):
# Atributes
my_object.time = time
my_object.name = name
my_object.wheels = wheels
if name is not None and wheels is not None:
print("It is expected the car {} will be built with {} wheels".format(name, wheels))
print(f"The car {my_object.name} was built succesfully")
else:
print("A car was built")
Delete an object
del my_car
Access a class property
my_car.name = 'Mustang'
print(f"This is an attribute of the car: {my_car.name}")
Execute a class method
my_car.my_method()
Delete a property
del my_car.name
Encapsulation
It consists of not allowing access to private methods and attributes from outside the class. To declare a method or attribute as private, start the name with a double underscore “__”, in this way you can only access these methods or attributes from inside the class
class cars_factory:
# Private method
__private_feature = True
def my_method(self):
print(f"This attribute is accessed only inside the class: {self.__private_feature}")
Inheritance
The parent class is indicated as an argument in the declaration of the child class. In the following example, the "mustang_factory" class inherits all the methods and properties of the parent class, without defining anything additional, so the "pass" keyword is used.
class mustang_factory(cars_factory):
pass
The child class can define its own methods and properties, so that if there is a coincidence of names, they will be overwritten, leaving the definition made in the child class. Also the child class can define its own __init__ function and within this call the __init__ class of the parent class, in order to maintain the inheritance of such function.
class mustang_factory(cars_factory):
def __init__(self, year, time, name=None, wheels=None):
# mustang_factory new atribute
self.year = year
# calling cars_factory.__init__()
cars_factory.__init__(self, time, name, wheels)
The “super()” function allows you to execute the inheritance of all the methods and properties of the parent class, in this case without pointing to the name of the parent class and without using the “self” keyword to execute the methods of the parent class
class mustang_factory(cars_factory):
def __init__(self, year, time, name=None, wheels=None):
# mustang_factory atributes
self.year = year
# calling cars_factory.__init__()
super().__init__(time, name, wheels)
Multiple inheritance
It is the ability to inherit attributes and methods from various classes, in the case of overridden methods, priority is given to the first class declared in the inheritance
class first_class():
def __init__(self):
print("I am the first class")
class second_class():
def __init__(self):
print("I am the second class")
class third_class(first_class, second_class):
def __init__(self):
print('I am the third class')
first_class.__init__(self)
second_class.__init__(self)
test = third_class()
Polymorphism
It refers to the ability to make a specific treatment to each object, according to the requirement.
class first_class():
def my_method(self):
print("I am the first class")
class second_class():
def my_method(self):
print("I am the second class")
class third_class():
def my_method(self):
print('I am the third class')
def polymorphism(object):
object.my_method()
test = third_class()
polymorphism(test)
#The class to use depends on the instance of the object
Thanks for reading :)
I invite you to continue reading other entries and visiting us again soon.