Functions
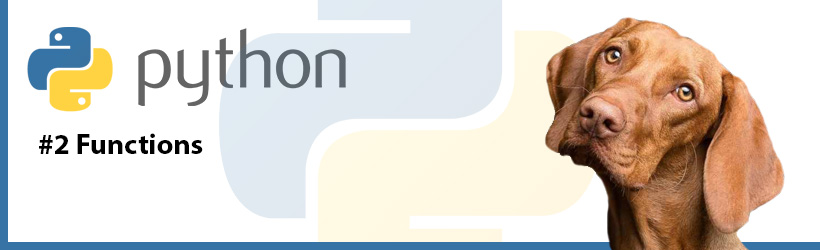
def my_function(param1, param2):
a = param1+param2 # code of the function
return a
#Calling to the function
result=my_function('param1','param2')
By default the parameters are taken in the order they are passed, but this order can be forced:
# Calling to the function
result = my_function(param2='param1', param1='param2')
Parameter passing by value
In Python, parameters are passed by value, except that the variable will retain changes only in cases where it is of a mutable data type. In case of using an immutable data type, the behavior is equivalent to passing parameters by reference.
# Using immutable data (int, float, str, bool, tuple, frozenset)
def my_function(value):
value = value+100
return value
value = 15
result = my_function(value)
print('The value of value in the function is: ' + str(result)) # 115
print('The value of global value is: ' + str(value)) # 15
# Using mutable data (list, dict, set)
def my_function(value):
value[1] = value[1]*100
return value
value = [1, 2, 3, 4, 9, 6, 7, 8, 9]
result = my_function(value)
print(result) # [1, 200, 3, 4, 9, 6, 7, 8, 9]
print(value) # [1, 200, 3, 4, 9, 6, 7, 8, 9]
Passing null parameters
The function can be executed in case that not all the defined parameters have been passed, since the missing parameters are defined by default as "None"
def null_values(x=None, y=None):
if y == None:
y = 0
return x+y
x = 5
y = 10
result = null_values(x, y) # result=15
print(result)
result = null_values(x) # result=5
print(result)
Passing parameters with default values
It is the same case above, but values other than "None" are set.
def other_values(x=20, y=100):
return x+y
x = 5
y = 10
result = other_values(x, y) # result=15
print(result)
result = other_values(x) # result=105
print(result)
Functions with variable number of arguments
In this case, the last argument must be preceded by * in case you want to handle the possible arguments in a list, or it can be preceded by ** in case you want to handle the possible arguments with a dictionary, where the keys will be the names of the parameters passed in the function call, and the values will be their corresponding equalities.
def several_args(a, b, *my_list):
print(a)
print(b)
print(my_list)
a = 'several'
b = 'args'
c = 'other'
d = 'other more'
several_args(a, b, c, d)
Should you handle variable arguments with a dictionary:
def several_args(a, b, **my_dict):
print(a)
print(b)
print(my_dict)
a = 'several'
b = 'args'
several_args(a, b, c='other', d='other more')
Recursive functions
def back(seconds):
seconds -= 1
if seconds >= 0:
print(seconds)
back(seconds)
else:
print('It finished')
back(5)
Lambda function
It is a function with a simplified syntax, also known as “on the go”, “on demand” or “on line”.
The lambda function can receive any number of parameters, but its procedure is limited to a single expression.
def calc(x, y): return x ** 2+y
result = calc(2, 3)
print(result) # 7
Filter function
It consists of applying a function that returns 'True/False' to a list. Then the filter function returns a new list with the values that generated the 'True' response.
values = [1, 2, 3, 4, 5, 6, 7, 8, 9]
even_numbers = list(filter(lambda x: x % 2 == 0, values))
print(even_numbers) # [2, 4, 6, 8]
Map function
The map function applies a function to each element of a sequence and returns a list with the result of applying the function to each element. If 'n' sequences are passed as parameters, the function will have to accept 'n' arguments. If any of the sequences is smaller than the others, the value passed to the function for positions greater than the size of that sequence will be None.
def square(n):
return n ** 2
l = [1, 2, 3]
l2 = list(map(square, l))
print(l) # [1, 2, 3]
print(l2) # [1, 4, 9]
Thanks for reading :)
I invite you to continue reading other entries and visiting us again soon.