Render HTML templates
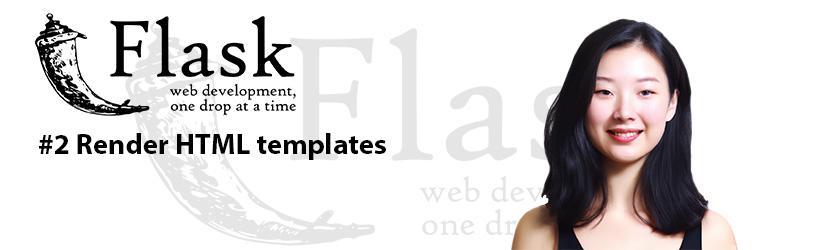
For this, the 'render_template' object is imported. By applying the function 'render_template' and sending as parameters to the file 'hello.html' and the variable 'name' and 'title', the file would look like this:
from flask import Flask # Importing Flask
from flask import render_template # Function for rendering templates
app = Flask(__name__)
# For every route must exist a function
@app.route('/')
def hello_world():
title = 'My web'
return render_template('hello.html', name='Fernando', title=title)
# File 'templates/hello.html' , Sending the var 'name' and 'title'
if __name__ == '__main__':
app.run(debug=True, port=80)
Note: If you want to save the HTML templates in another directory, you must indicate it in the app definition.
from flask import Flask # Importing Flask
from flask import render_template # Function for rendering templates
app = Flask(__name__, template_folder="new_templates")
File ‘templates/hello.html’:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>{{title}}</title>
</head>
<body>
<h1>Hello {{name}}</h1>
</body>
</html>
You can also send all the data in a dictionary:
def hello_world():
data = {
'name': 'Fernando',
'title': 'My web'
}
return render_template('hello.html', data=data)
And in the view the dictionary values are retrieved as follows:
{{data.title}}
Dynamic web pages
Flask employs the Jinja2 templating engine, below is an example to send parameters from the main server file to the '.html' file
Main file:
from flask import Flask # Importing Flask
from flask import render_template # Function for rendering templates
app = Flask(__name__)
# For every route must exist a function
@app.route('/') # This indicates the route start on the root
def index(): # Function which define the root route
name = 'Fernando' # Parameters to send to the view
course = 'Python'
premium = True
list_of_courses = ['Python', 'C#', 'Javascript', 'PHP']
return render_template('index.html', username=name, # Here we add the vars that want to send to the view
current_course=course,
premium=premium,
list_of_courses=list_of_courses)
if __name__ == '__main__':
app.run(debug=True, port=80)
Archivo .html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>course of {{current_course}}</title>
</head>
<body>
<!--Print a variable: {{var}}-->
<h1>My name is {{username}}</h1>
<p>I am using Flask</p>
<!--If-else: {% if %}{% else %}{% endif %}-->
{% if premium %}
<p>The course is Premium</p>
{% else %}
<p>The course is Free</p>
{% endif %}
{% if list_of_courses %}
<p>
Enjoy our courses:
</p>
<ul>
<!--For: {% for %}{% endfor %}-->
{%for item in list_of_courses%}
<li>{{item}}</li>
{% endfor %}
</ul>
{% endif %}
</body>
</html>
Some examples in jinja2
When working with Jinja2 we must keep in mind 3 important elements: 1. Variables {{ variable }} 2. Instructions {% instruction %} 3. Comments {# comments #}
Example 1: Using variables
<!--Uso de variables-->
<h2>Hola {{ username }} </h2>
<p>
Te encuentras en el curso {{ course.title }}
</p>
Example 2: Conditional if
<!--Condicional if-->
{% if user_is_admin %}
<a href="{{ url_for('admin') }}">admin</a>
{% else %}
<p>No cuentas con los permisos suficientes</p>
{% endif %}
Example 3: Conditional if
<!--Condicional if-->
{% if user_is_admin and user.permission_level == 5 %}
<a href="{{ url_for('admin') }}">admin</a>
{% endif %}
Example 4: for loop
<!--Ciclo for-->
<ul>
{% for val in [1,2,3,4,5,6,7,8,9] %}
<li> {{ val }} </li>
{% endfor %}
</ul>
Within the loop we can access different attributes of the loop object:
1. index: Current interaction. Value starts at 1 2. index0: Current iteration. The value starts at 0 (Ideal if we want to replicate the behavior of the enumerate function) 3. first: True value if we are in the first iteration. 4. last: True value if we are in the last iteration. 5. length: Number of iterations.
Example 5: for loop with attributes of the loop object
<ul>
{% for val in [1,2,3,4,5,6,7,8,9] %}
<li> {{ loop.index0 }} - {{ val }} </li>
{% endfor %}
</ul>
Example 6: Sending functions to the html template
Python-file:
def addition(val1, val2):
return val1 + val2
def addition_template():
return render_template('suma.html', val1=10,
val2=30, funcion= addition)
html-file:
<p>
The addition of {{ val1 }} + {{ val2 }} is : {{ funcion(val1, val2) }}
</p>
Thanks for reading :)
I invite you to continue reading other entries and visiting us again soon.