Dynamic URL
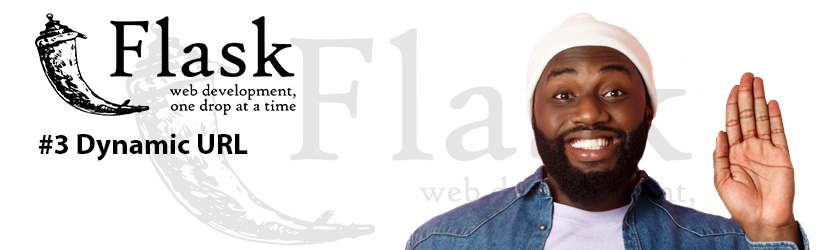
Functions must be defined for each URL or each URL pattern, as shown in the example:
@app.route('/users/<name>') #
def users(name): # The function receives the parameters
return 'Hello ' + name
As long as the requested URL matches the pattern 'localhost:port/users/
Here is an example with multiple parameters in the URL:
@app.route('/users') # No parameters
@app.route('/users/<name>') # <1 parameter>
@app.route('/users/<name>/<lastname>') # <2 parameters>
@app.route('/users/<name>/<lastname>/<int:age>') # <3 parameters> The third must be integer to match with the route
def users(name="generic name", lastname="generic lastname", age=0): # The function receives the parameters
return 'Hello ' + name + ' ' + lastname + '. Your age is ' + str(age)
In the example above, the route is executed upon receiving a request with a URL of the type:
‘localhost:port/users/fernando/carrillo/34’
Another way to receive parameters through the URL is with the structure:
dominio/section?parameter1=first-parameter& parameter2=second-parameter
In this case, the request method is used:
from flask import Flask, request
While in the route function we have the following:
@app.route('/params')
def params():
first_param = request.args.get('first-param', 'first param not found')
second_param = request.args.get('second-param', 'second param not found')
return 'The first param is: {} and the second param is: {}'.format(first_param, second_param)
Thanks for reading :)
I invite you to continue reading other entries and visiting us again soon.