General concepts Docker
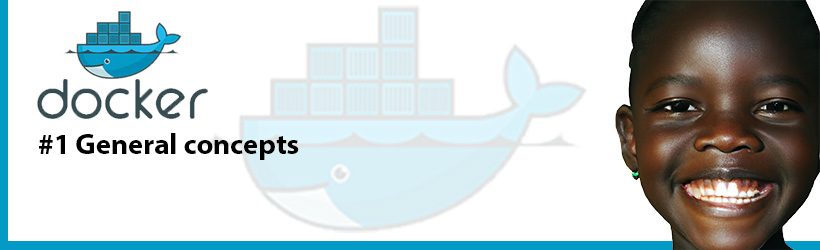
What is Docker?
Docker is a platform and technology that allows you to develop, deploy, and run applications inside lightweight, isolated environments called containers. Containers are a form of virtualization that packages an application and its dependencies, including libraries and runtime, in a consistent and portable manner. This enables developers to build and ship applications along with their required components, ensuring that they run consistently across various environments, from development to testing to production.
Important concepts
Containers
Containers are isolated environments that encapsulate an application and its dependencies, ensuring consistency and portability across different systems. They use the host operating system's kernel, which makes them lightweight and efficient compared to traditional virtual machines.
Images
Docker images are read-only templates that define the environment and instructions for setting up an application. Images can be created from a Dockerfile, which is a text file containing a set of instructions for building an image. Images serve as the basis for creating and running containers.
Docker Engine
Docker Engine is the core component of Docker that manages containers, images, and networking. It includes a server, a REST API, and a command-line interface for interacting with Docker.
Docker Compose
Docker Compose is a tool for defining and managing multi-container applications. It uses a YAML file to define the services, networks, and volumes required for an application to run. This simplifies the process of managing complex applications composed of multiple containers.
Docker Hub
Docker Hub is a cloud-based repository where you can find and share Docker images. It provides a convenient way to access and distribute pre-built images, saving time and effort in setting up software environments.
Orchestration
Docker Swarm and Kubernetes are orchestration tools that help manage and scale containerized applications in production environments. They provide features such as load balancing, auto-scaling, and service discovery to ensure high availability and resilience.
Now let's use some commands
Images
Download latest image
This allows to download from Docker Hub the image tagged as latest. The next example shows how to download the latest official Python image:
docker pull python
Download specific version
This allows to download from Docker Hub an specific image. The next example shows how to download the official Python image tagged as python:3.12.0b4-alpine3.18:
docker pull python:3.12.0b4-alpine3.18
See downloaded images
The next command permits to list the downloaded images and to see some features from them, like tag, id and size.
You can notice according to the image, the size can vary a lot.
docker images
Delete image
The next command (docker image rm <image-id>) permits to delete an image pointing out the image's id. At the picture, we can see 2 Python and Postgres images, and we are going to delete both latest tagged images.
docker image rm <image-id>
Build image
After being working with an image, we can create a new image for not loosing (and sharing) the settings we made.
docker build -t new_image_name .
-t: Tag (to name the image)
Rename an image (add tag) to upload to Docker Hub
docker tag <image-id> (or <image-name>) user_docker/image_name:version
Networks
View configured networks
docker network ls
Create a docker network
docker network create net_name
Delete a network
docker network rm net_name
Containers
Create container
For creating a new container we must indicate which image is the container based on.
docker create <image-id> (This generates an id)

Another option
docker container create <image-id>
Create container by assigning name
docker create --name <container-name> <image-id>
Create container assigning port and name
docker create -p:27017:27017 --name container_name imagen_base
docker create -p:host_port:container_port
Activate the container
docker start <container-id> (or <container-name>)
Show active container
docker ps
Stop a container
docker stop container_id
Show all containers
docker ps -a
Delete container
docker rm container_id (or container_name)
Stop and delete the container
docker rm -f <container-id> (or <container-id>)
View container logs
docker logs container_name
Continuously view container logs
docker logs --follow container_name (Ctrl + c to exit)
docker logs -f container_name
Run a command in the container
docker exec -it container_name sh (this command is to enter the container OS console)
Docker run
Docker run actions
1. Verify the existence of the image
2. If it does not exist, the download
3. Create the container
4. Start the container
5. Show logs in follow mode
docker run image_name
Additional parameters
-d -> “Detached mode”, do not show the logs of the activated server
-p 3000:5000 -> Define host ingress port (eg: 3000) and container port (eg: 5000)
-network network_name -> Internal network the container is on
-e variable1=value_1 -> Environment variable to start the container
-it -> Interactive mode, to interact with the container system
-w /folder -> Sets the working directory
--network network_name
--mount type=volume,src=volume_name,target=path -> Copy the contents of the container "path" to the respective directory on the host so that the changes are persistent, the location given to these files on the host is determined by Docker (named volume).
-v volume_name:/path -> Short form to declare a named volume
--mount type=bind,src="$(pwd)",target=/path -> Mirror the content of src, which is a directory on the host to the “path” directory on the container and vice versa (bind volume).
Docker run setup complete
docker run --name container_name -p:host_port:container_port --network network_name -d -it -w /folder -v volumen_name:/path --mount type=bind,src="$(pwd)",target=/path -e variable1=value_1 -e variablen=value_n image_name
Volumes
It is the function of defining a route in the container whose content will be reflected in the host.
There are two types of volumes, “named volume” and “bind volume”. In the name volume the path in the container is set but Docker chooses where it will save that data on the host, while in the bind volume the path is set in both systems and the changes of each one will be reflected in the other.
Create volume of type “named volume”
docker volume create volume_name
Thanks for reading :)
I invite you to continue reading other entries and visiting us again soon.