Conversion to militar format time
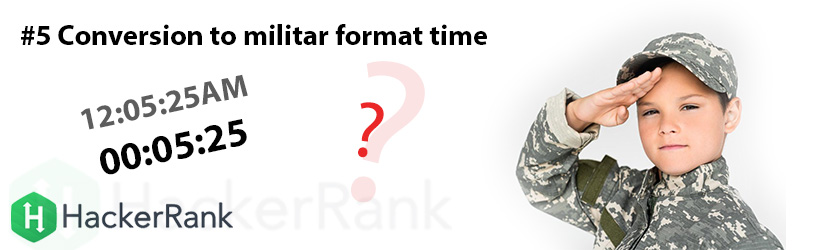
Given a time in 12-hour AM/PM format, convert it to military (24-hour) time.
Note: - 12:00:00AM on a 12-hour clock is 00:00:00 on a 24-hour clock.
- 12:00:00PM on a 12-hour clock is 12:00:00 on a 24-hour clock.
Sample Input
07:05:45PM
Sample Output
19:05:45
This kind of exercise is very common for learning the programming basis, on this occasion I took it from HackerRank.
My solution
#
# Complete the 'time_conversion' function below.
#
# The function is expected to return a STRING.
# The function accepts STRING s as parameter.
#
def time_conversion(time12:str)->str:
'''
Convert from format 12H to format 24H.
Take the string with the structure '07:05:45PM' and
return a string with the structure '19:05:45'
Parameters
----------
time12 : str
Time in 12H format.
Return
------
time24:str.
Time in 24H format.
Examples
--------
input '07:05:45PM'
output '19:05:45'
input '12:05:45AM'
output '00:05:45'
'''
time24=time12[:-2]
if time12[-2:] == 'PM' and time12[:2] != '12':
time24 = str(int(time12[:2])+12)+time12[2:-2]
elif time12[-2:] == 'AM' and time12[:2]== '12':
time24 = '00'+ time12[2:-2]
return time24
if __name__ == '__main__':
s = '07:05:45PM'
result = time_conversion(s)
print(result)
Explanation
I get started considering the cases when the unique change is taking out 'AM/PM' at the end of the string.
time24=time12[:-2]
Later, I evaluate the case of 'PM' but after 12H : '01:05:45PM' -> '13:05:45', then, from '01 PM' to '11 PM' it is necessary add 12 to the hour value:
if time12[-2:] == 'PM' and time12[:2] != '12':
time24 = str(int(time12[:2])+12)+time12[2:-2]
Finally, I evaluate the case of 'AM' but exactly at 12H : '12:05:45AM' -> '00:05:45', on this case, it is necessary to subtract 12 from the hour value:
elif time12[-2:] == 'AM' and time12[:2]== '12':
time24 = '00'+ time12[2:-2]
Other points to clarify:
time12[-2:] : It returns the last two characters of time12.
time12[:2] : It returns the first two characters of time12.
time12[2:-2]: It returns time12 but removing the first two and the last two characters.
Thanks for reading :)
I invite you to continue reading other entries and visiting us again soon.