Consuming APIs in Python using the library
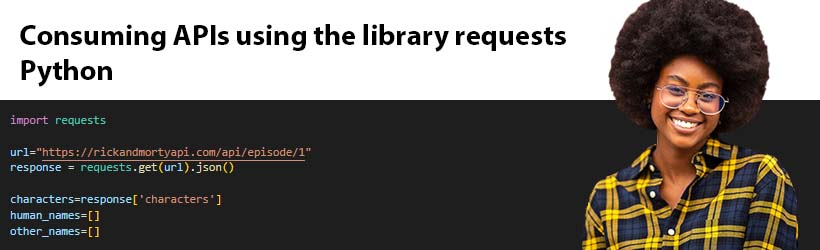
Install "requests" library
The first step I allways recommend is: Set a virtual enviroment
path-to-the-project>python -m venv env
In the last entry, 'env' is the directory that will be created to host the Virtual Environment
Now you need to activate it, you move to 'env/Scripts/' directory using the terminal:
path-to-the-project>cd env/Scripts
Finally, introduce the command 'activate' and the word '(env)' will appear at the beginning of the terminal line:
path-to-the-project\env\Scripts>activate
(env) path-to-the-project\env\Scripts>
Go back to your original directory:
(env) path-to-the-project\env\Scripts>cd..\..
(env) path-to-the-project>
Now we can install the library requests:
pip install requests
Example #1
In this first example, we are going to consume the API from https://gorest.co.in/, I recommend you to check the available endpoints and the instructions to consume them.
Let's bring the users from the endpoint https://gorest.co.in/public/v2/users:
import requests
url='https://gorest.co.in/public/v2/users'
response = requests.get(url)
if response.status_code==200:
response=response.json()
print(response)
else:
print("The error code received is {}".format(response.status_code))
It the endpoint is not available in that moment, we will receive an error code, e.g.:
The error code received is 522
On another hand, if everthing run ok, the status_code must be 200, and we can print the users, not before converting the response to JSON format. The print in the terminal would be something like:
[{'id': 1188507, 'name': 'Bhaanumati Rana', 'email': 'bhaanumati_rana@dibbert.test', 'gender': 'female', 'status': 'active'}, {'id': 1188506, 'name': 'Dhana Achari Jr.', 'email': 'dhana_achari_jr@gerhold-cruickshank.example', 'gender': 'female', 'status': 'active'}, {'id': 1188505, 'name': 'Gov. Diksha Achari', 'email': 'diksha_achari_gov@dibbert-veum.example', 'gender': 'male', 'status': 'active'}, {'id': 1188504, 'name': 'Devesh Varma', 'email': 'varma_devesh@graham-wiza.test', 'gender': 'female', 'status': 'active'}, {'id': 1188503, 'name': 'Dev Jha I', 'email': 'jha_i_dev@altenwerth-hauck.test', 'gender': 'female', 'status': 'inactive'}, {'id': 1188502, 'name': 'Buddhana Bhattacharya', 'email': 'buddhana_bhattacharya@douglas.test', 'gender': 'male', 'status': 'active'}, {'id': 1188501, 'name': 'Arnesh Varrier', 'email': 'arnesh_varrier@ledner.test', 'gender': 'male', 'status': 'active'}, {'id': 1188500, 'name': 'Lila Somayaji', 'email': 'somayaji_lila@klocko-ward.test', 'gender': 'female', 'status': 'active'}, {'id': 1188499, 'name': 'Pres. Bhanumati Somayaji', 'email': 'bhanumati_somayaji_pres@white-raynor.example', 'gender': 'male', 'status': 'active'}, {'id': 1188498, 'name': 'Rev. Aanjaneya Deshpande', 'email': 'aanjaneya_deshpande_rev@klocko.example', 'gender': 'female', 'status': 'inactive'}]
But this format is not friendly to read, let's use another complement that helps us to print the JSON data prettier.
Install the library "pprint":
pip install pprint
Now if we use pprint the above code must change like this:
import requests
from pprint import pprint
url='https://gorest.co.in/public/v2/users'
response = requests.get(url)
if response.status_code==200:
response=response.json()
pprint(response)
else:
print("The error code received is {}".format(response.status_code))
Now the answer should look like this:
[{'email': 'bhaanumati_rana@dibbert.test',
'gender': 'female',
'id': 1188507,
'name': 'Bhaanumati Rana',
'status': 'active'},
{'email': 'dhana_achari_jr@gerhold-cruickshank.example',
'gender': 'female',
'id': 1188506,
'name': 'Dhana Achari Jr.',
'status': 'active'},
{'email': 'diksha_achari_gov@dibbert-veum.example',
'gender': 'male',
'id': 1188505,
'name': 'Gov. Diksha Achari',
'status': 'active'},
{'email': 'varma_devesh@graham-wiza.test',
'gender': 'female',
'id': 1188504,
'name': 'Devesh Varma',
'status': 'active'},
{'email': 'jha_i_dev@altenwerth-hauck.test',
'gender': 'female',
'id': 1188503,
'name': 'Dev Jha I',
'status': 'inactive'},
{'email': 'buddhana_bhattacharya@douglas.test',
'gender': 'male',
'id': 1188502,
'name': 'Buddhana Bhattacharya',
'status': 'active'},
{'email': 'arnesh_varrier@ledner.test',
'gender': 'male',
'id': 1188501,
'name': 'Arnesh Varrier',
'status': 'active'},
{'email': 'somayaji_lila@klocko-ward.test',
'gender': 'female',
'id': 1188500,
'name': 'Lila Somayaji',
'status': 'active'},
{'email': 'bhanumati_somayaji_pres@white-raynor.example',
'gender': 'male',
'id': 1188499,
'name': 'Pres. Bhanumati Somayaji',
'status': 'active'},
{'email': 'aanjaneya_deshpande_rev@klocko.example',
'gender': 'female',
'id': 1188498,
'name': 'Rev. Aanjaneya Deshpande',
'status': 'inactive'}]
Now we can access to the items and apply conditionals to filter them, for example, in the next code we print just the users which gender is 'female' and status is 'inactive':
import requests from pprint import pprint url='https://gorest.co.in/public/v2/users' response = requests.get(url) if response.status_code==200: response=response.json() for user in response: if user['gender']=='female' and user['status']=='inactive': pprint(user) else: print("The error code received is {}".format(response.status_code))The answer should be something like:
{'email': 'jha_i_dev@altenwerth-hauck.test',
'gender': 'female',
'id': 1188503,
'name': 'Dev Jha I',
'status': 'inactive'}
{'email': 'aanjaneya_deshpande_rev@klocko.example',
'gender': 'female',
'id': 1188498,
'name': 'Rev. Aanjaneya Deshpande',
'status': 'inactive'}